Showing
- b_asic/signal.py 24 additions, 32 deletionsb_asic/signal.py
- b_asic/signal_flow_graph.py 233 additions, 171 deletionsb_asic/signal_flow_graph.py
- b_asic/signal_generator.py 13 additions, 13 deletionsb_asic/signal_generator.py
- b_asic/simulation.py 37 additions, 27 deletionsb_asic/simulation.py
- b_asic/special_operations.py 28 additions, 12 deletionsb_asic/special_operations.py
- examples/threepointwinograddft.py 2 additions, 0 deletionsexamples/threepointwinograddft.py
- examples/twotapfirsfg.py 6 additions, 6 deletionsexamples/twotapfirsfg.py
- pyproject.toml 3 additions, 3 deletionspyproject.toml
- setup.py 9 additions, 11 deletionssetup.py
- test/baseline/test_draw_matrix_transposer_4.png 0 additions, 0 deletionstest/baseline/test_draw_matrix_transposer_4.png
- test/baseline/test_draw_process_collection.png 0 additions, 0 deletionstest/baseline/test_draw_process_collection.png
- test/fixtures/interleaver-two-port-issue175.p 0 additions, 0 deletionstest/fixtures/interleaver-two-port-issue175.p
- test/fixtures/resources.py 7 additions, 7 deletionstest/fixtures/resources.py
- test/fixtures/signal_flow_graph.py 36 additions, 4 deletionstest/fixtures/signal_flow_graph.py
- test/test_operation.py 26 additions, 43 deletionstest/test_operation.py
- test/test_process.py 24 additions, 0 deletionstest/test_process.py
- test/test_resources.py 21 additions, 30 deletionstest/test_resources.py
- test/test_schedule.py 120 additions, 107 deletionstest/test_schedule.py
- test/test_sfg.py 182 additions, 157 deletionstest/test_sfg.py
- test/test_sfg_generators.py 6 additions, 16 deletionstest/test_sfg_generators.py
This diff is collapsed.
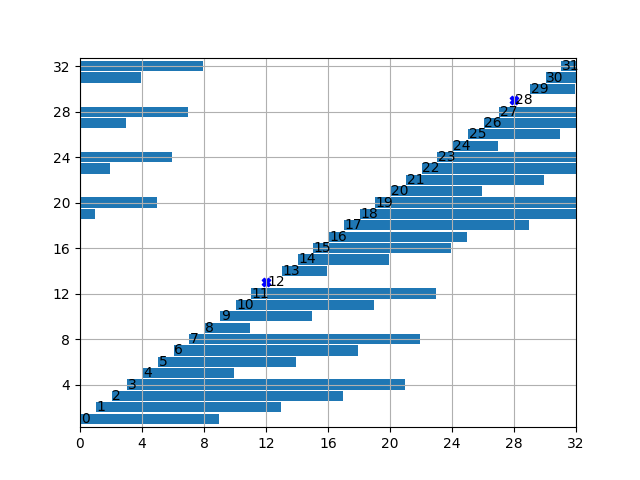
| W: | H:
| W: | H:
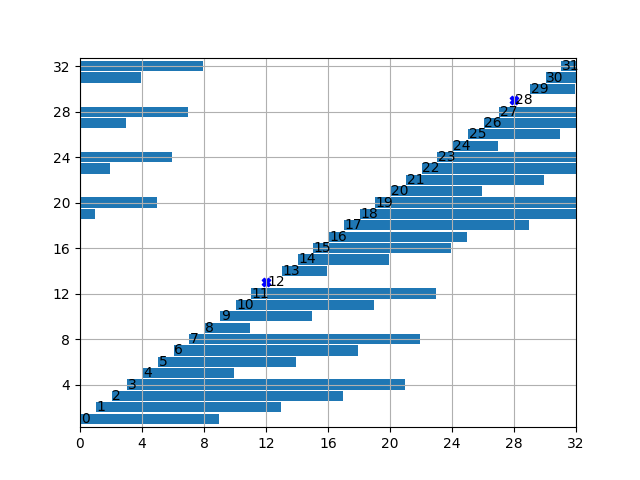
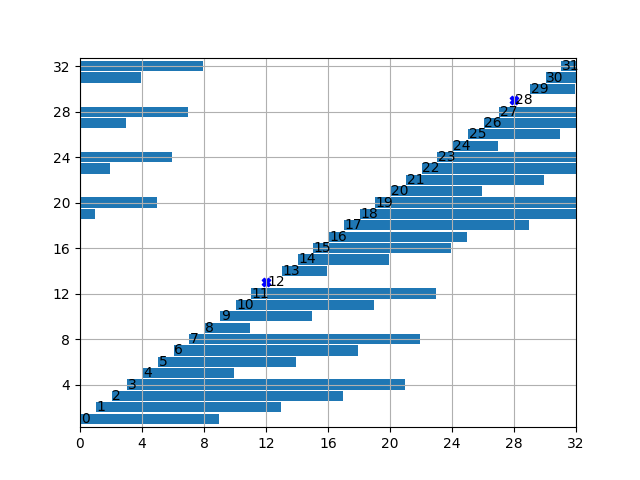
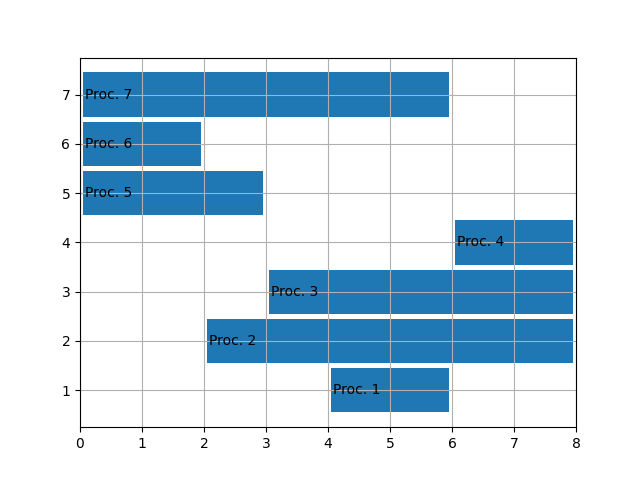
| W: | H:
| W: | H:
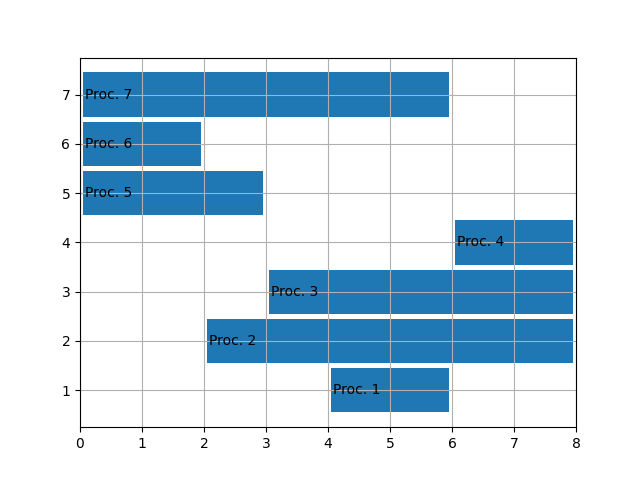
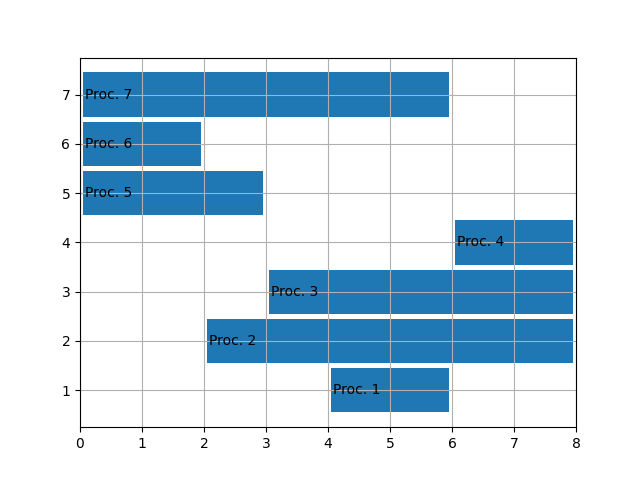
File added
This diff is collapsed.