Commits on Source (2)
-
Mikael Henriksson authored
-
Showing
- b_asic/resources.py 19 additions, 7 deletionsb_asic/resources.py
- b_asic/signal_flow_graph.py 3 additions, 2 deletionsb_asic/signal_flow_graph.py
- test/baseline/test_draw_matrix_transposer_4.png 0 additions, 0 deletionstest/baseline/test_draw_matrix_transposer_4.png
- test/test_operation.py 1 addition, 1 deletiontest/test_operation.py
- test/test_sfg.py 22 additions, 0 deletionstest/test_sfg.py
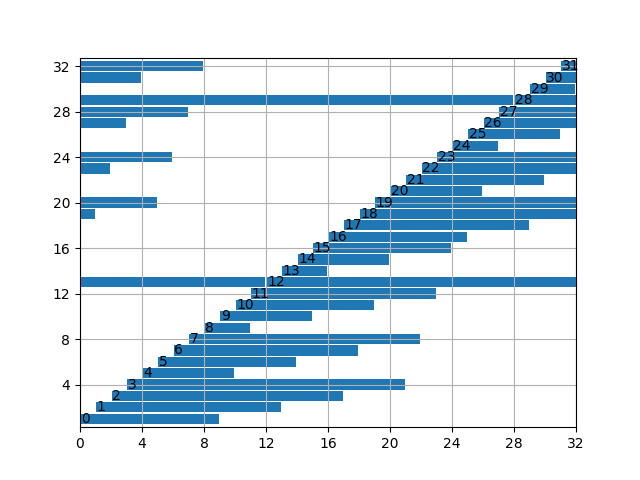
| W: | H:
| W: | H:
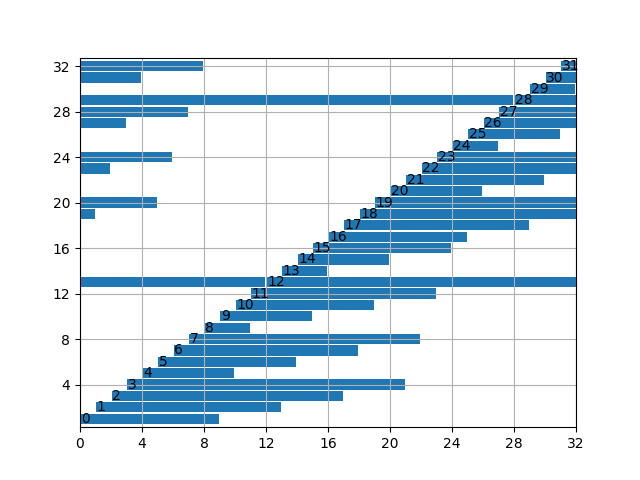
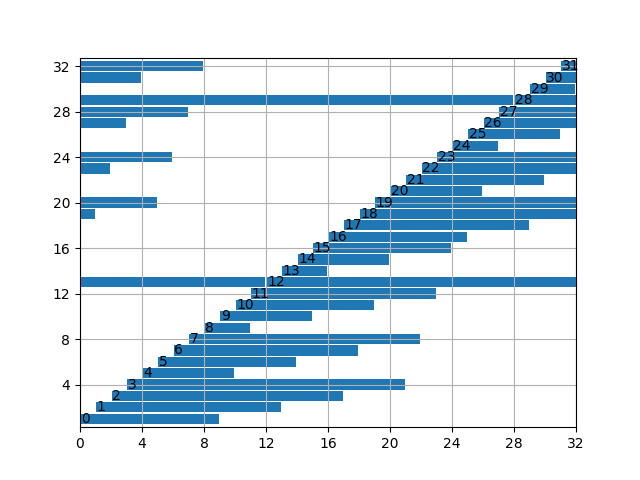