-
- Downloads
Fix #163 and add documentation
Showing
- b_asic/schedule.py 89 additions, 14 deletionsb_asic/schedule.py
- b_asic/scheduler_gui/main_window.py 1 addition, 1 deletionb_asic/scheduler_gui/main_window.py
- b_asic/signal_flow_graph.py 48 additions, 17 deletionsb_asic/signal_flow_graph.py
- b_asic/signal_generator.py 6 additions, 0 deletionsb_asic/signal_generator.py
- examples/secondorderdirectformiir.py 1 addition, 1 deletionexamples/secondorderdirectformiir.py
- examples/threepointwinograddft.py 1 addition, 1 deletionexamples/threepointwinograddft.py
- logo.svg 3 additions, 3 deletionslogo.svg
- test/baseline/test__get_figure_no_execution_times.png 0 additions, 0 deletionstest/baseline/test__get_figure_no_execution_times.png
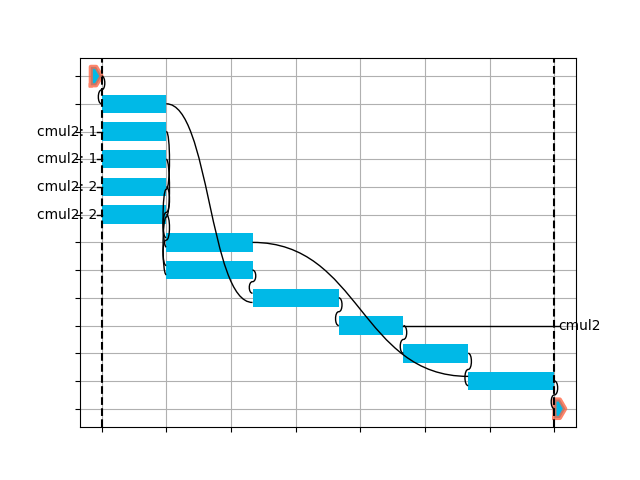
| W: | H:
| W: | H:
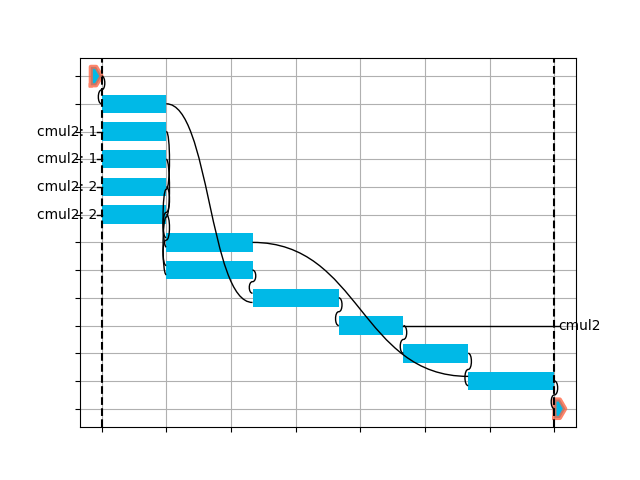
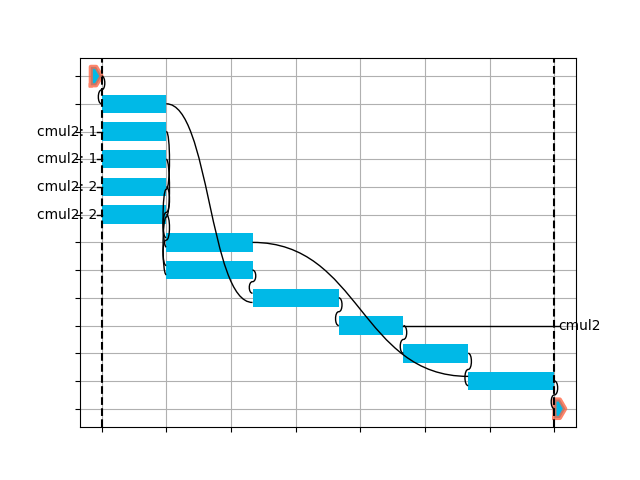